Program in C Language to implement calculator that can perform Addition, Subtraction, Multiplication, Division and find remainder using Modulus operator
CODE
#include<stdio.h>
int main(){
float n1,n2,res;
int num1,num2,res1;
char op;
printf("Enter the Operator : ");
scanf("%c",&op);
if(op=='+'||op=='-'||op=='*'||op=='/'){
printf("Enter First Operand : ");
scanf("%f",&n1);
printf("Enter Second Operand : ");
scanf("%f",&n2);
if(op=='+'){
res=n1+ n2;
}
if(op=='-'){
res=n1- n2;
}
if(op=='*'){
res=n1 *n2;
}
if(op=='/'){
if(n2==0)
printf("Division by zero is not possible");
if(n2!=0)
res= n1/n2;
}
if(!(op=='/'&&n2==0)){
printf("%f %c %f = %f",n1,op,n2,res);
}
}
if(op=='%')
{
printf("Enter First Operand : ");
scanf("%d",&num1);
printf("Enter Second Operand : ");
scanf("%d",&num2);
res1= num1%num2;
printf("%d %c %d = %d",num1,op,num2,res1);
}
if(!(op=='+'||op=='-'||op=='*'||op=='/'||op=='%'))
printf("Sorry. Wrong opeartor");
printf("\n");
return 0;
}
First we input operator in variable of character datatype op so that we can decide whether we have to store values in int or float. Since modulus can only use int data type.
Now if operator given by user is among ‘+’ , ‘- ‘, ‘*’, ‘/’ we will input both values in float variables namely n1, n2 and store result in float variable res. While calculating division we also have to check if n2 ==0, since division by zero is not possible.
if(op=='/'){
if(n2==0)
printf("Division by zero is not possible");
if(n2!=0)
res= n1/n2;
}
Now while printing the output, we again have to check if op is equal to ‘/’ and n2 equal to 0, then we don’t have to print any thing since we have already displayed and error message else we have to print the result. That’s why we have used the inverse of the condition
if(!(op=='/'&&n2==0)){
printf("%f %c %f = %f",n1,op,n2,res);
}
Now if operator given is modulus then we will store the values in variables of int datatype namely num1, num2 and will store result in int variable res1.
if(op=='%'){
printf("Enter First Operand : ");
scanf("%d",&num1);
printf("Enter Second Operand : ");
scanf("%d",&num2);
res1= num1%num2;
printf("%d %c %d = %d",num1,op,num2,res1);
}
Now if the operator inputted is none of ‘+’, ‘-‘, ‘*’, ‘/’ and ‘%’ then we can not calculate the result so we will display a error message “Sorry. Wrong opeartor”
if(!(op=='+'||op=='-'||op=='*'||op=='/'||op=='%'))
printf("Sorry. Wrong opeartor");
OUTPUT
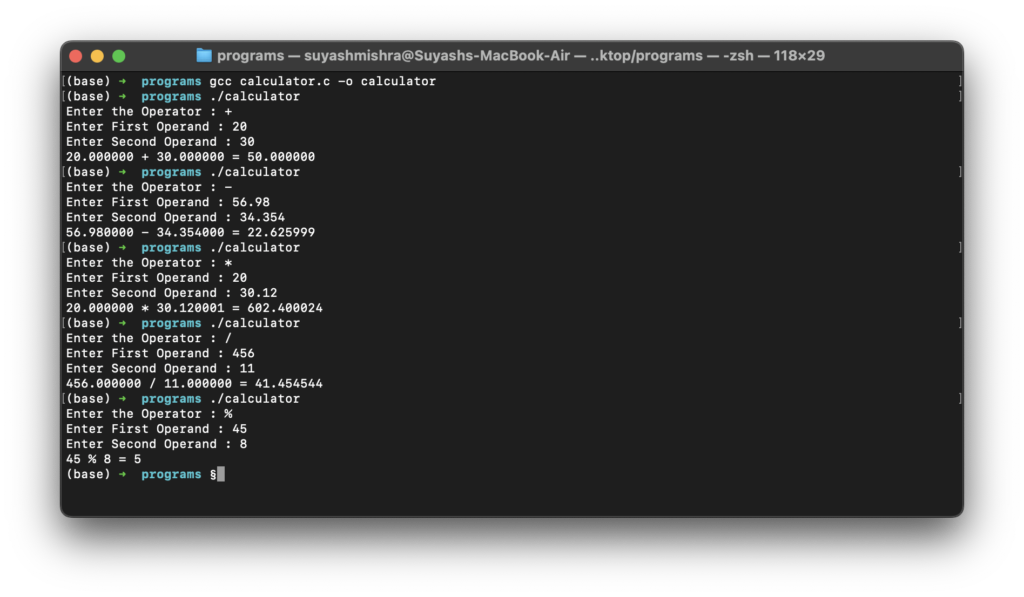
NOTE: While using float we can get some weird (not accurate answers) like 56.980000 – 34.354000 = 22.625999 . float
s are not capable of storing exact values for every possible number (infinite numbers between 0-1 therefore impossible). Assigning 0.00002 to a float will have a different but really close number due to the implementation which is what you are experiencing. Precision decreases as the number grows.
Thank you for reading this blog.